Bot Saves Princess problem is Hackerrank is the kind of game that I used to play a lot when I was a child. But I have never thought of writing code to save some Princess Peach who is trapped in some corners of a square grid. Well, it was much more challenging than to play.
Now, let’s take a look at the input format and understand what we need to do.
Input format:
—–
–m–
—–
p—-
—–
The first line contains an odd integer N (3 <= N < 100) denoting the size of the grid. The bot position is denoted by ‘m’ and the princess position is denoted by ‘p’. We need to print the moves to rescue the princess. The valid moves are LEFT or RIGHT or UP or DOWN. So, for this example, the expected output is :
DOWN
LEFT
DOWN
LEFT
The Hackerrank Compiler doesn’t like it if your solution says DOWN-DOWN-LEFT-LEFT or LEFT-LEFT-DOWN-DOWN. So, let’s start with the code part and find a solution for which Hackerrank scores you 13.9.
Code1:
# Enter your code here. Read input from STDIN. Print output to STDOUT stdin <- file('input') open(stdin) input <- suppressWarnings(read.table(stdin, sep=" ",stringsAsFactors = FALSE)); close(stdin) #Read the first line n <- as.integer(input$V1[1]) i <- 1 for( i in 1: n){ j <- 1 inputline <- unlist(strsplit(input$V1[i+1],"")) inputline <- unlist(strsplit(inputline,"")) for (j in 1:n){ if (inputline[j] == "p"){ rp=i cp=j } else if (inputline[j] == "m"){ rm=i cm=j } } } x=rp-rm y=cp-cm
Let me explain what I am doing in the above piece of code:
1. I am reading the size of the grid i.e. “n” and opening two for loops of size n
2. Within the Loop, I am reading the position of “m” and “p” and thus tracking the row and column position of the Bot and princess.
At this point, Let’s check the position values:
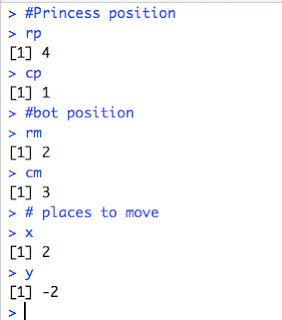
So, the princess is at [4,1] and the bot is at [2,3]. Now, if we consider these two points in a two-dimensional plane, x denotes positive 2 since we need to go 2 places up and y denotes negative 2 since we need to go two places left.
Now, let’s write logic to print the UP or DOWN, LEFT or RIGHT.
displayPathtoPrincess <- function(n, x, y){ while (x!= 0 | y!= 0){ if(x > 0){ cat('DOWN') cat("\n") x = x - 1 if(y > 0){ cat('RIGHT') cat("\n") y = y - 1 } else if(y < 0){ cat('LEFT') cat("\n") y = y + 1 } } else if (x < 0){ cat('UP') cat("\n") x = x + 1 if(y > 0){ #cat('RIGHT', sep="\n") cat('RIGHT') cat("\n") y = y - 1 } else if(y < 0){ cat('LEFT') cat("\n") y = y + 1 } } else { if(y > 0){ cat('RIGHT') cat("\n") y = y - 1 } else if(y < 0){ cat('LEFT') cat("\n") y = y + 1 } } } } displayPathtoPrincess(n,x,y)
I am running the while loop till I reach the princess position. My simple logic is x greater than 0 means we need to move down till I reach the row where the Princess is and vice versa if x is less than 0, we need to move up till we reach the princess row. Similarly, if y is greater than 0 that means, we need to move Right and if it is less than 0 we need to move till we reach the princess column. Does that make any sense? Well, if you write the code and play the game in the Hackerrank compiler step by step then it will be crystal clear. Now, let’s take the look at the Function output:
Well, that was the expected output and solving the challenge was Fun!
Thank You for reading!
0