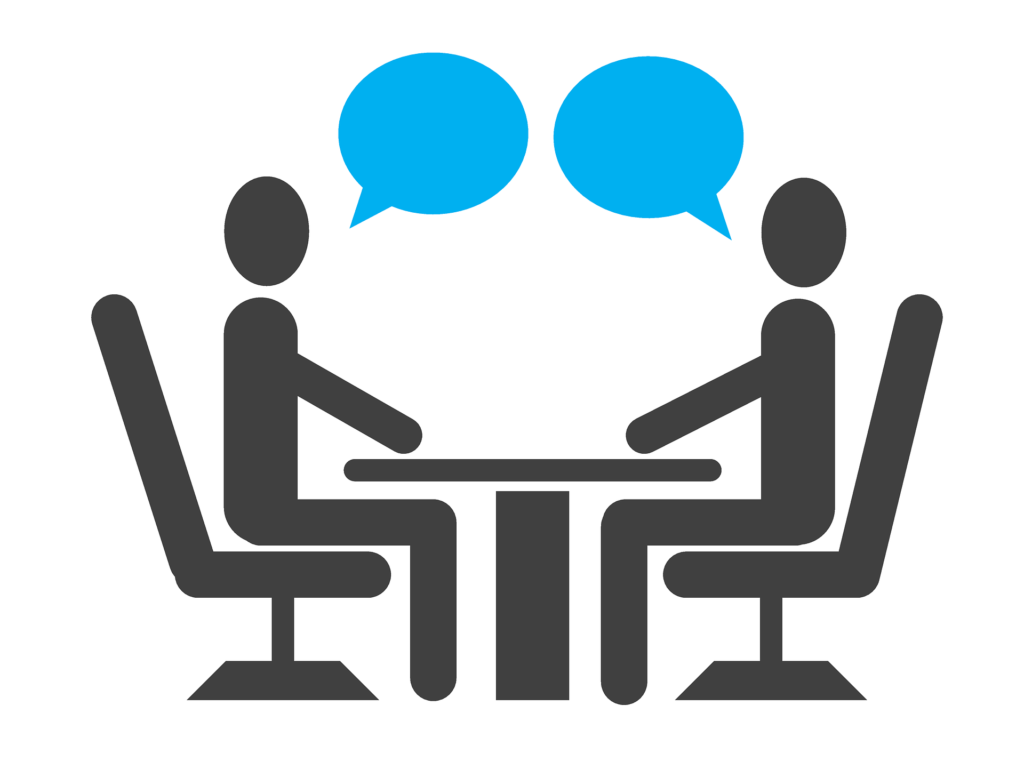
This post is the repository of some python interview questions. The questions will range from basic theory questions to answering the outputs of some code. I have posted before on Python interview questions, but this post is more vigorous, and I am trying to keep it as a one-stop solution to brush up on the Python skills.
My others posts on python interview preparation are as follows:
2o Basic Programming Problems and Solutions in Python
Python Programming Questions for Data Science Interview – Part I
Python Theory Interview Questions:
1. What are the key features of Python?
Interpreted Language: No need to compile before running
Object Oriented: Allows definition of classes along with inheritance
Open Source and free: Anyone can use, create and contribute to its development
Portable: Easy to run same code on Windows or MAC or Linux
Large Standard Library: A large collection of modules and functions to support
Dynamically Typed Language: No need to declare the variables type
2.What is the difference between a list and a tuple?
Lists | Tuple |
---|---|
Lists are mutable. | Tuples are immutable. |
Lists consume more memory | Tuples consume less memory as compared to lists |
Lists are slowers than tuples. | Tuples are faster than lists. |
Syntax: a_list = [1,0,’Apple’, True] | Syntax: a_tuple = (1,90,’Banana’, False) |
3. How to convert a list into a tuple and vice versa?
A list can be converted to a tuple using the tuple() function and to convert a tuple to a list we can use the list() function like below:
weekdays = ['sun','mon','tue','wed','thu','fri','sat'] weekdays_tup = tuple(weekdays) print("Tuple: ", weekdays_tup) print(type(weekdays_tup)) weekdays_list = list(weekdays_tup) print("List: ",weekdays_list) print(type(weekdays_list))
4. How to convert a list to a String?
To convert a list into a string, we can use the Join() method which joins all the elements and return as a string.
weekdays = ['sun','mon','tue','wed','thu','fri','sat'] week_str = ' '.join(weekdays) print(week_str) print(type(week_str))
5. How do you debug a Python script?
- Putting Print commands – Add print commands to print different variables and display information. But the drawback in this method is we need to do lots of changes in the code and rerun.
- Use python built-in debugger pdb – with the below command we can debug a python script
$ python3 -m pdb python-script.py
6. What is PYTHONPATH?
PYTHONPATH is an environment variable to locate a module whenever it is imported.
7. What is <Yield> Keyword in Python?
The yield statement suspends the function’s execution and sends a value back to the caller, but retains enough state to enable the function to resume where it is left off. The difference between the Return and Yield is that “return” sends a specific value back to its caller whereas Yield can produce and return a sequence of values over time rather than computing them at once and sending them back like a list.
Example:
def test_yield(index): weekdays = ['sun','mon','tue','wed','thu','fri','sat'] yield weekdays[index] index = index + 2 yield weekdays[index]
Now, let’s call the above function to see the output:
day = test_yield(0) print(next(day)) print(next(day))
8. What is a negative index in Python?
In Python, a negative index reads the elements from the end of an array or list.
weekdays = ['sun','mon','tue','wed','thu','fri','sat'] print(weekdays[-1]) print(weekdays[-5])
9. What is Enumerate() Function in Python?
In Python, enumerate() method adds a counter to an iterable object. That means when we use enumerate(), the function gives back two loop variables:
- The count of the current iteration
- The value of the item at the current iteration
weekdays = ['sun','mon','tue','wed','thu','fri','sat'] print(list(enumerate(weekdays)))
We can also use it in a for loop like below:
weekdays = ['sun','mon','tue','wed','thu','fri','sat'] for count, value in enumerate(weekdays): print(count, value)
10. How to count the occurrences of a particular element in the list?
In Python, we can count the occurrences of an individual element in a list by using a count() function.
weekdays = ['sun','mon','fri','thu','mon','sat','mon','tue','wed','thu','fri','sat'] weekdays.count('mon')
11. What is __int__?
_init_ is a reserved method or constructor in Python. This method is automatically called to allocate memory when a new object/ instance of a class is created. All classes have the __init__ method.
class Rectangle: def init(self, length, breadth): self.length = length self.breadth = breadth def get_area(self): return self.length * self.breadth r = Rectangle(160, 120) print("Area of Rectangle: %s sq units" % (r.get_area()))
12. What is NumPy array?
NumPy arrays can create an N-dimensional homogeneous array in python and element-wise operation is possible like LISTS. NumPy arrays are more compact, efficient and faster than Lists.
import numpy as np a = np.array([1, 2, 3]) print(a)
13. How can you create an Empty NumPy Array In Python?
We can create an Empty NumPy Array in two below ways in Python:
Method1:
import numpy numpy.array([])
Method2:
numpy.empty(shape=(0,0))
14. What is self in Python?
Self is an instance of a class. In Python, this is explicitly included as the first parameter. If we don’t provide it, it will cause an error.
class check_self: def find_self(): print("Inside find_self")
The above code generates the below error:
TypeError Traceback (most recent call last) <ipython-input-39-2c3e4dfa121e> in <module> 1 check = check_self() ----> 2 check.find_self() TypeError: find_self() takes 0 positional arguments but 1 was given
class check_self: def find_self(self): print("Inside find_self")
15. How to generate random numbers in Python?
We can generate random numbers using the below functions in Python.
1. random() – This command returns a floating point number, between 0 and 1.
2. randint(X, Y) – This command returns a random integer between the values given as X and Y.
3. Uniform(X, Y) – This command returns a floating point number between the values given as X and Y
import random print(random.random()) print(random.randint(1, 10)) print(random.uniform(1, 10))
16. How do you Concatenate Strings in Python?
In Python, strings can be concatenated using a “+” sign.
print('Practice' + ' ' + 'Python')
17. What is a lambda function?
In Python, a function without a name (anonymous) is lambda function.
double = lambda x: x * 2 print(double(5)
18. How can you randomize the items of a list in place in Python?
In Python, the items of a list can be shuffled using a shuffle() function.
from random import shuffle x = ['today', 'we', 'are', 'practicing', 'Python'] print(x) shuffle(x) print(x)
19. What is pickling and unpickling?
Pickle module accepts any Python object and converts it into a string representation and dumps it into a file by using the dump function, this process is called pickling. While the process of retrieving original Python objects from the stored string representation is called unpickling.
import pickle #initializing data to be stored in db wild_animals = {'name': 'lion','food_habit': 'carnivores','habitat': 'jungle' } domestic_animals = {'name': 'cow','food_habit': 'herbivores','habitat': 'farm' } database db = {} db['wild_animals'] = wild_animals db['domestic_animals'] = domestic_animals #For pickling b = pickle.dumps(db) # type(b) gives #For unpickling myEntry = pickle.loads(b) print(myEntry)
20. What are Lists and Dictionary comprehensions?
Lists and Dictionary comprehensions are a concise way to create new lists and dictionaries respectively.
List Comprehension Example:
nums = [2,3,4,7,5,12] number_list = [x * 2 for x in nums if x % 2 == 0] print(number_list)
Dictionary Comprehension Example:
create list of fruits fruits = ['apple', 'mango', 'bananas','cherry'] dict comprehension to create dict with fruit name as keys fruits_dict = {f:len(f) for f in fruits} print(fruits_dict)
21. What is break, continue and pass in python?
Break | The break statement terminates the loop immediately and the control flows to the statement after the body of the loop. |
Continue | The continue statement terminates the current iteration of the statement, skips the rest of the code in the current iteration and the control flows to the next iteration of the loop. |
Pass | The pass statement is used when a statement is required syntactically but you do not want any command or code to execute. It is like null operation. |
##Break example for i in range(1, 11): # If i is equals to 6, break if i == 6: break else: # otherwise print the value of i print("Break", i) ##Continue example for i in range(1, 11): # If i is equals to 6, continue to next iteration if i == 6: continue print(i) else: # otherwise print the value of i print('Continue',i) ##Pass example for i in range(1, 11): # If i is equals to 6,continue to next iteration without printing if i == 6: pass print("pass executed") else: # otherwise print the value of i print('Pass', i)
22. What are docstrings in Python?
Docstrings are the documentation strings that appear right after the definition of a function, method class or module within triple quotes. They are used to document the code. These docstrings can be accessed using _doc_ attribute.
def square(n): '''Takes in a number n, returns the square of n''' return n**2 print(square._doc_)
23. What are Python Modules?
The Files containing Python codes are referred to as Python Modules. This code can either be classes, functions, or variables and saves the programmer’s time by providing the predefined functionalities when needed. It is a file with the .py extension, containing an executable code.
Commonly used built-in modules are listed below:
- os
- sys
- data time
- math
- random
- JSON
24. What do *args and **kwargs mean?
*args: The special syntax *args in function definitions in python is used to pass a variable number of arguments to a function. It is used to pass a non-keyworded, variable-length argument list.
def myFun(*argv): for arg in argv: print (arg) myFun('Python','is','fun.') myFun('Hello','Python!')
**kwargs: The special syntax **kwargs in function definitions in python is used to pass a keyworded, variable-length argument list. A keyword argument is where we can provide a name to the variable as we pass it into the function.
def myFun(**kwargs): for key, value in kwargs.items(): print ("%s == %s" %(key, value)) Driver code myFun(first ='Python', mid ='is', last='fun')
25. How can files be deleted in Python?
To delete a file we can import OS module and use the os.remove() function.
import os os.remove("file_name")
26. What is the difference between / and // operators in Python?
“/” is a division operator and returns the quotient value.
“//” is known as the floor division operator and used to return only the value of the quotient before the decimal part.
For Example, 10/3 will return 3.33 and 10 // 3 is 3.
27. How do we identify and deal with missing values in DataFrames?
The isnull() and isna() functions are used to identify the missing values in a DataFrame.
The numerical missing values can be filled in with zero or mean of all the available values.
df[‘col_name’].fillna(0) df[‘col_name’] = df[‘col_name’].fillna((df[‘col_name’].mean()))
28. How to create a dataframe from lists?
import pandas as pd import numpy as np list1 = ['Apple', 'Orange', 'Grapes','Blueberry','Banana','Melon'] list2 = ['Red','Orange',np.nan, 'Blue','Yellow', np.nan] df = pd.DataFrame() df["fruits"] = list1 df["color"] = list2 df
29. Give the above dataframe drop all rows having Nan.
df.dropna(inplace = True) df
30. How can you insert an element at a given index in Python?
An element can be inserted in a list at a given position using the insert() function.
list1 = [ 0,1, 2, 3, 4, 5, 6, 7 ] #insert 10 at 6th index list1.insert(6, 10) list1
31. How will you remove duplicate elements from a list?
The most popular way by which the duplicates are removed from the list is by convering it into a set and then convert it back to a list.
my_list = [1,1,2,3,2,2,4,5,6,2,1] my_final_list = set(my_list) print(list(my_final_list))
32. What is “Call by Value” in Python?
In pass-by-value, we pass values of the parameter to the function. If any kind of change is done to those parameters inside the function, those changes are not reflected back in the actual parameters.
33. What is “Call by Reference” in Python?
In pass-by-reference, we pass reference of parameters to the function. If any changes are made to those parameters inside function those changes are get reflected back to the actual parameters.
34. Is Python “Call by Value” or “Call By Reference” ?
In Python, neither “Call by Value” nor “Call By Reference” are applicable, rather the values are sent to functions by means of an object reference. Now, if an object is immutable(int, float, string, tuple) then the modified value is not available outside the function. And if the object is mutable (list, set, dict) then the modified value is available outside the function.
def test(string):
string = "python & java" print("Inside Function:", string)
string = "python"
test(string)
print("Outside Function:", string)
#####
def add_more(list):
list.append(50)
print("Inside Function", list)
mylist = [10,20,30,40]
add_more(mylist)
print("Outside Function:", mylist)
35. What is the purpose of “end” in Python?
Python’s print() function always prints a newline in the end. The print() function accepts an optional parameter known as the ‘end.’ By default, its value is ‘\n’. But, we can change the end character in a print statement with the value of our choice using this parameter.
print("You are doing great") print("You are doing great", end = ' ') print('and have some fun')
Now, in interviews, there are some questions where we need to guess the outputs of a few lines of code. So, let’s try to guess the output of some python problems without actually solving it!
Guess the output:
Problem: 36
names = ['Chris', 'Jack', 'John', 'Daman']
print((names[-1][-1]))
Answer: n
Problem: 37
import array
a = [1, 2, 3]
print (a[-3])
print (a[-2])
print (a[-1])
Answer:
1 2 3
Problem: 38
"Hello World"[::-1]
Answer: ‘dlroW olleH’
Problem: 39
a = [1, 3, 2] print(a * 2)
Answer:
[1, 3, 2, 1, 3, 2]
Problem: 40
list1 = ['s', 'r', 'a', 's'] list2 = ['o','e','t','o'] ["".join([i, j]) for i, j in zip(list1, list2)]
Answer: [‘so’, ‘re’, ‘at’, ‘so’]
Problem: 41
names1 = ['Amir', 'Bear', 'Charlton', 'Daman'] names2 = names1 names3 = names1[:] names2[0] = 'Alice' names3[1] = 'Bob' sum = 0 for ls in (names1, names2, names3): if ls[0] == 'Alice': sum += 1 if ls[1] == 'Bob': sum += 10 print(sum)
Answer: 12
Problem: 42
r = lambda q: q * 2 s = lambda q: q * 3 x = 2 x = r(x) x = s(x) x = r(x) print (x)
Answer: 24
Problem: 43
count = 1 def doThis(): global count for i in (1, 2, 3): count += 1 doThis() print (count)
Answer: 4
Problem: 44
class Acc: def init(self, id): self.id = id id = 555 acc = Acc(111) print (acc.id)
Answer: 111
Problem: 45
for i in range(2): print(i) for i in range(4,6): print(i)
Answer:
0 1 4 5
Problem: 46
def extendList(val, list=[]): list.append(val) #print("Inside extendList: " ,list) return list list1 = extendList(10) list2 = extendList(123,[]) list3 = extendList('a') print ("list1 = %s" % list1) print ("list2 = %s" % list2) print ("list3 = %s" % list3)
Answer:
list1 = [10, 'a'] list2 = [123] list3 = [10, 'a']
Problem: 47
list = ['a', 'b', 'c', 'd', 'e'] print (list[10:])
Answer: []
Problem: 48
str = 'pdf csv json' print(str.split())
Answer:
['pdf', 'csv', 'json']
Problem: 49
str = 'lEaRn pYtHoN' print(str.title())
Answer:
Learn Python
Python provides the title() method to convert the first letter in each word to capital format while the rest turns to Lowercase.
Problem: 50
S = [['him', 'sell'], [90, 28, 43]] S[0][1][1]
Answer: e
There can be a lot of possible questions in a Python Interview. This post is just a few of them. But, let’s start practicing until we master it! Well, my best wishes are there for you!
Let’s rock the next Python Interview!!!
Thank You!
1